Firebase is a popular mobile platform powered by Google. It helps you to quickly develop high-quality, enterprise-grade applications and grow your business.
RudderStack supports Firebase as a mobile device mode to which you can seamlessly send your customer data for analytics.
Getting started
Before configuring Firebase as a destination in RudderStack, verify if the source platform is supported by Firebase by referring to the table below:
Connection Mode | Web | Mobile | Server |
---|---|---|---|
Device mode | - | Supported | - |
Cloud mode | - | - | - |
Once you have confirmed that the source platform supports sending events to Firebase, follow these steps:
- From your RudderStack dashboard, add a source. Then, from the list of destinations, select Firebase.
- Assign a name to the destination and click Next.
Connection settings
Connect this destination to your Android/iOS/Unity/React Native source. You should then see the following screen:
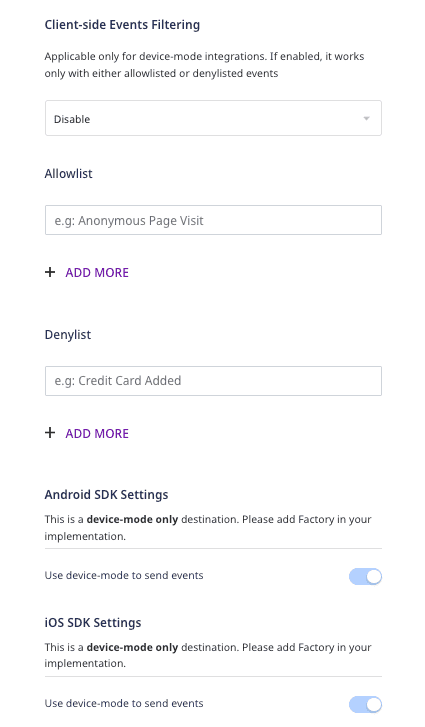
- Client-side Events Filtering: Refer to the Client-side Event Filtering guide for more information on this setting.
As this is a device mode-only integration, click Next to complete the destination setup.
Adding device mode integration
Depending on your platform of integration, follow the steps below to add Firebase to your project:
- Register your mobile app in the Firebase console.
- Once you have successfully created the app in the Firebase console, you will be prompted to download the
google-services.json
file. - Copy this file in the
app
folder of your project. It contains all the necessary information about the project and the integration. - Add the
classpath
underdependencies
to your project levelbuild.gradle
, as shown:buildscript {repositories {google()}dependencies {// add this lineclasspath 'com.google.gms:google-services:4.3.3'}} - Now, you can add the
plugins
anddependencies
to yourapp/build.gradle
file, as shown:apply plugin: 'com.android.application'apply plugin: 'com.google.gms.google-services' - Then, add the
mavenCentral
repository, as shown:repositories {mavenCentral()} - Add the RudderStack-Firebase SDK extension along with the
core
SDK underdependencies
, as shown:implementation 'com.rudderstack.android.sdk:core:1.+'implementation 'com.rudderstack.android.integration:firebase:2.+'//Firebaseimplementation platform('com.google.firebase:firebase-bom:28.4.2')implementation 'com.google.firebase:firebase-analytics' - Next, add the necessary
permissions
underAndroidManifest.xml
, as shown:<uses-permission android:name="android.permission.INTERNET" /><uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> - Finally, change the SDK initialization in your
Application
class, as shown:val rudderClient = RudderClient.getInstance(this,<SOURCE_WRITE_KEY>,RudderConfig.Builder().withDataPlaneUrl(<DATA_PLANE_URL>).withFactory(FirebaseIntegrationFactory.FACTORY).build())
- Register your app in the Firebase console. It will then prompt you to download the
GoogleServices-Info.plist
file. - Add the file to the root of your XCode project.
- Go to your
Podfile
and add theRudder-Firebase
extension along with the core SDK using the following code:pod 'Rudder-Firebase', '~> 2.0.5' - After adding the dependency followed by the
pod install
command, you can add the imports to yourAppDelegate.m
file, as shown:#import "RudderFirebaseFactory.h" - Finally, change the SDK initialization, as shown in the following snippet:RSConfigBuilder *builder = [[RSConfigBuilder alloc] init];[builder withDataPlaneUrl:<DATA_PLANE_URL>];[builder withFactory:[RudderFirebaseFactory instance]];[builder withLoglevel:RSLogLevelDebug];[RSClient getInstance:<WRITE_KEY> config:[builder build]];
Rudder-Firebase
version 2.0.5
is tested and fully compatible with Firebase/Analytics
version 8.15.0
.- Add Firebase as a destination in the RudderStack dashboard.
- Register your app in the Firebase console. It will then prompt you to download the
GoogleServices-Info.plist
file. - Download and place this file in your project.
- Install
RudderFirebase
(available through CocoaPods) by adding the following line to yourPodfile
:pod 'RudderFirebase', '~> 1.0.0' - Run the
pod install
command. - Then, import the SDK depending on your preferred platform:import RudderFirebase@import RudderFirebase;
- Next, add the imports to your
AppDelegate
file under thedidFinishLaunchingWithOptions
method, as shown:RSConfig *config = [[RSConfig alloc] initWithWriteKey:WRITE_KEY];[config dataPlaneURL:DATA_PLANE_URL];[[RSClient sharedInstance] configureWith:config];[[RSClient sharedInstance] addDestination:[[RudderFirebaseDestination alloc] init]];let config: RSConfig = RSConfig(writeKey: WRITE_KEY).dataPlaneURL(DATA_PLANE_URL)RSClient.sharedInstance().configure(with: config)RSClient.sharedInstance().addDestination(RudderFirebaseDestination())
- Register your project in the Firebase Console. Currently, RudderStack supports only Android and iOS platforms for Unity.
- After adding the project, Firebase will prompt you to download the
google-services.json
for Android andGoogleServices-Info.plist
for iOS. - Add those two files to your project's
Assets
folder. - Integrate the RudderStack core SDK with your project. For more information, refer to the Unity SDK documentation.
- Download and import the Firebase Unity SDK and follow the instructions to add the Firebase SDK (specifically,
FirebaseAnalytics.unitypackage
) to your project. - Download the RudderStack Firebase Extension from the GitHub page and import it in your project.
- Attach the
RudderPreferbs.prefab
file fromRudder
to your mainGameObject
- Finally, change the SDK initialization using the following code snippet:// Build your configRudderConfigBuilder configBuilder = new RudderConfigBuilder().WithEndPointUrl(<DATA_PLANE_URL>).WithFactory(RudderFirebaseIntegrationFactory.GetFactory());// get instance for RudderClientRudderClient rudderClient = RudderClient.GetInstance(<SOURCE_WRITE_KEY>,configBuilder.Build());
- Register your Android and iOS applications in the Firebase console.
- Once you have successfully created the applications in the Firebase console, you will be prompted to download the
google-services.json
andGoogleServices-Info.plist
files. - Add the RudderStack React Native SDK to your app by referring to the React Native SDK documentation.
- Add the RudderStack-Firebase React Native module to your app using the following command:npm install @rudderstack/rudder-integration-firebase-react-native// OR //yarn add @rudderstack/rudder-integration-firebase-react-native
- Next, import the module you added above and add it to your SDK initialization code as shown:import rudderClient from '@rudderstack/rudder-sdk-react-native';import firebase from "@rudderstack/rudder-integration-firebase-react-native";const config = {dataPlaneUrl: <DATA_PLANE_URL>,trackAppLifecycleEvents: true,withFactories: [firebase]};rudderClient.setup(<SOURCE_WRITE_KEY>, config);
- Navigate to your app's
android
folder and follow the steps below:- Copy the
google-services.json
file in theapp
folder of your Android project. This file contains all the necessary information about the project and the integration. - Add the
classpath
underdependencies
to your project levelbuild.gradle
file, as shown:buildscript {repositories {google()}dependencies {// add this lineclasspath 'com.google.gms:google-services:4.3.3'}} - Once you have completed the steps above, you can add the plugins and dependencies to your
app/build.gradle
file, as shown:apply plugin: 'com.android.application'apply plugin: 'com.google.gms.google-services' - Then, add the necessary permissions under
AndroidManifest.xml
, as shown:<uses-permission android:name="android.permission.INTERNET" /><uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
- Copy the
- Finally, navigate to your app's
iOS
folder and follow these steps:- Install all the required pods using the
pod install
command. - Add the
GoogleServices-Info.plist
file to the root of your XCode project.
- Install all the required pods using the
- Add the following dependency to the
dependencies
section of yourpubspec.yaml
file.rudder_integration_firebase_flutter: ^1.0.1 - Run the below command to install the dependency added in the above step:flutter pub get
- Import the
RudderIntegrationFirebaseFlutter
in your application where you are initializing the SDK.import 'package:rudder_integration_firebase_flutter/rudder_integration_firebase_flutter.dart'; - Change the initialization of your
RudderClient
as shown:final RudderController rudderClient = RudderController.instance;RudderConfigBuilder builder = RudderConfigBuilder();builder.withFactory(RudderIntegrationFirebaseFlutter());rudderClient.initialize(<WRITE_KEY>, config: builder.build(), options: null); - Navigate to your app's
android
folder and follow the below steps:- Copy the
google-services.json
file in theapp
folder of your Android project. This file contains all the necessary information about the project and the integration. - Add the
classpath
underdependencies
to your project levelbuild.gradle
file, as shown:buildscript {repositories {google()}dependencies {// add this lineclasspath 'com.google.gms:google-services:4.3.3'}} - Add
mavenCentral()
as a repository underbuildscript.repositories
andallprojects.repositories
to your project levelbuild.gradle
file, as shown:buildscript {repositories {google()// should be addedmavenCentral()}dependencies {classpath 'com.google.gms:google-services:4.3.3'}}allprojects {repositories {google()// should be addedmavenCentral()}} - Next, add the plugins and dependencies to your
app/build.gradle
file, as shown:apply plugin: 'com.android.application'apply plugin: 'com.google.gms.google-services' - Add the necessary permissions under
AndroidManifest.xml
, as shown:<uses-permission android:name="android.permission.INTERNET" /><uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
- Copy the
- Finally, navigate to your app's
iOS
folder and add theGoogleServices-Info.plist
file to the root of your XCode project.
Identify
The identify
call sets the userId
through the setUserId
method from FirebaseAnalytics
. RudderStack sets the other user properties from RudderTraits
to Firebase using the setUserProperty
method.
RudderStack ignores age
, gender
, and interest
, as these properties are reserved by Google.
[[RSClient sharedInstance] identify:@"test_user_id" traits:@{@"foo": @"bar", @"foo1": @"bar1", @"email": @"test@gmail.com", @"key_1" : @"value_1", @"key_2" : @"value_2" }];
Track
RudderStack's track
events are mapped to the standard Firebase events wherever possible.
Event mapping
RudderStack maps the following events to the standard Firebase events:
RudderStack Event | Firebase Event |
---|---|
Payment Info Entered | add_payment_info |
Product Added | add_to_cart |
Product Added to Wishlist | add_to_wishlist |
Application Opened | app_open |
Checkout Started | begin_checkout |
Order Completed | purchase |
Order Refunded | refund |
Products Searched | search |
Cart Shared | share |
Product Shared | share |
Product Viewed | view_item |
Product List Viewed | view_item_list |
Product Removed | remove_from_cart |
Product Clicked | select_content |
Promotion Viewed | view_promotion |
Promotion Clicked | select_promotion |
Cart Viewed | view_cart |
The following Firebase events are not mapped to any RudderStack event:
number_of_nights
number_of_rooms
number_of_passengers
origin
destination
start_date
end_date
travel_class
item_list_name
creative_slot
location_id
transaction_id
screen_class
RudderStack passes all the event-related properties to Firebase. The nested values in the properties are converted to JSON using GSON.
Property mapping
RudderStack maps the following event properties to the standard Firebase properties:
RudderStack property | Firebase property |
---|---|
category | item_category |
cart_id ,product_id | item_id |
share_via | method |
query | search_term |
revenue , value , total | value |
currency | currency |
tax | tax |
shipping | shipping |
coupon | coupon |
name | name , promotion_name |
quantity | quantity |
price | price |
payment_method | payment_type |
list_id | item_list_id |
promotion_id | promotion_id |
creative | creative_name |
affiliation | affiliation |
Along with mapping the above list of the standard property names, RudderStack exhibits the following behavior:
- Converts the event names to the lower case.
- Trims the whitespaces at the start and the end.
- Replaces a space with an underscore.
Firebase enforces a strict event name length limit of 40 characters. RudderStack takes a substring of 40 characters (from the beginning) if the event names exceed this permitted value.
A sample track
call sent from the iOS SDK is shown below:
[[RSClient sharedInstance] track:@"simple_track_with_props" properties:@{ @"key_1" : @"value_1", @"key_2" : @"value_2"}];
Screen
The screen
method lets you record whenever a user sees their mobile screen along with any optional properties about the viewed screen.
A sample screen
call is as shown:
[[RSClient sharedInstance] screen:@"Home Screen" properties:@{ @"Width" : @"13" }];
Reset
The reset
method resets the user identification.
A sample reset
call is shown below:
[[RSClient getInstance] reset];
RudderClient.getInstance()?.reset()
Debugging
You can check the events and their properties in the Firebase DebugView. To enable it for Android, run the following command from your terminal:
$ adb shell setprop debug.firebase.analytics.app <your_package_name>
For iOS, specify the following in your command line argument in XCode:
-FIRAnalyticsDebugEnabled
FAQ
How do I disable automatic screen tracking while using the RudderStack SDKs?
- For Android, nest the following setting within the
<application>
tag of yourAndroidManifest.xml
file:
<meta-data android:name="google_analytics_automatic_screen_reporting_enabled" android:value="false" />
- For iOS, set
FirebaseAutomaticScreenReportingEnabled
toNO
(Boolean) in yourInfo.plist
.
For more information, refer to the Firebase documentation.
Contact us
For more information on the topics covered on this page, email us or start a conversation in our Slack community.